joyful
TIP 12: 객체 펼침 연산자로 정보를 갱신하라 본문
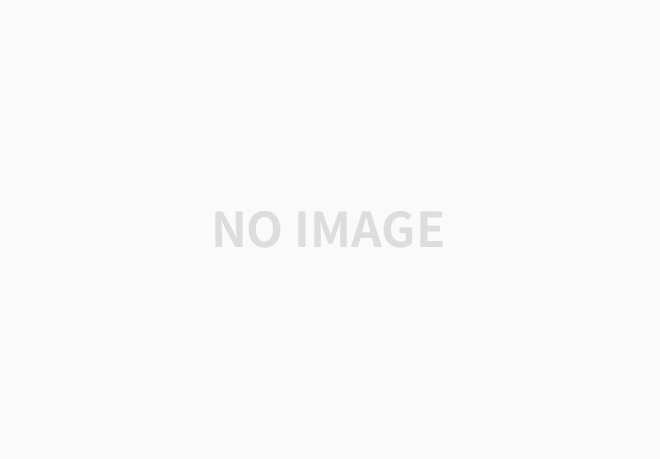
이번 팁에서는 Object.assign()의 이점을 객체 펼침 연산자의 간단한 문법으로 대체하는 법을 알아보자.
객체 펼침 연산자
- 배열 펼침 연산자와 작동법이 비슷하다.
- 키-값 쌍을 목록에 있는 것처럼 반환한다.
- 새로운 정보를 펼침 연산자의 앞이나 뒤에 쉽게 추가할 수 있다.
- 배열 펼침 연산자와 마찬가지로 독립적으로 사용할 수 없고 객체에 펼쳐지게 해야 한다.
function updateBookYear() {
const book = {
title: 'Reasons and Persons',
author: 'Derek Parfit',
};
const update = { ...book, year: 1984 };
// { title: 'Reasons and Persons', author: 'Derek Parfit', year: 1984}
}
function updateBookTitle() {
const book = {
title: 'Reasons and Persons',
author: 'Derek Parfit',
};
const update = { ...book, title: 'Reasons & Persons' };
// { title: 'Reasons & Persons', author: 'Derek Parfit' }
}
배열 펼침 연산자와 다른 점은 동일한 키에 서로 다른 값을 추가하면
어떤 값이든 가장 마지막에 선언된 값을 사용한다는 점이다. (title이 'Reasons & Persons'로 바뀐 점!)
이전 팁에서 작성했던 함수를 다시 작성해보자.
Object.assign()을 이용해서 정보를 추가하거나 갱신하는 방법은 아래와 같다.
const defaults = {
author: '',
title: '',
year: 2017,
rating: null,
};
const book = {
author: 'Joe Morgan',
title: 'Simplifying JavaScript',
};
const updated = Object.assign({}, defaults, book);
위의 코드에서 객체 펼침 연산자를 사용하면 다음과 같다.
const defaults = {
author: '',
title: '',
year: 2017,
rating: null,
};
const book = {
author: 'Joe Morgan',
title: 'ES6 Tips',
};
const bookWithDefaults = { ...defaults, ...book };
// {
// author: 'Joe Morgan',
// title: 'ES6 Tips',
// year: 2017,
// rating: null,
// }
하지만 Object.assign()과 마찬가지로 중첩 객체의 깊은 병합 문제가 펼침 연산자에서도 동일하게 발생한다.
이 문제는 다음과 같이 해결할 수 있다.
원래 코드:
const employee2 = Object.assign(
{},
defaultEmployee,
{
name: Object.assign({}, defaultEmployee.name),
},
);
해결 코드:
function deepMerge() {
const defaultEmployee = {
name: {
first: '',
last: '',
},
years: 0,
};
const employee = { ///펼침 연산자로 정리
...defaultEmployee,
name: {
...defaultEmployee.name,
},
};
employee.name.first = 'joe';
return [defaultEmployee.name.first, employee.name.first];
}
'자바스크립트 코딩의 기술' 카테고리의 다른 글
TIP 13: 맵으로 명확하게 키-값 데이터를 갱신하라 (1) | 2023.11.17 |
---|---|
TIP 11: Object.assign()으로 조작 없이 객체를 생성하라 (1) | 2023.11.11 |
TIP 10: 객체를 이용해 정적인 키-값을 탐색하라 (2) | 2023.11.07 |
TIP 9: 펼침 연산자로 정렬에 의한 혼란을 피하라 (2) | 2023.11.04 |
TIP 8: push() 메서드 대신 펼침 연산자로 원본 변경을 피하라 (0) | 2023.11.04 |